Problem Statement
We needed to set a default value for the field_assigned_user_email field on the node_reservation_request_form. The challenge was to locate the correct array path to this field within the form structure, as it was not immediately apparent where the field was nested.
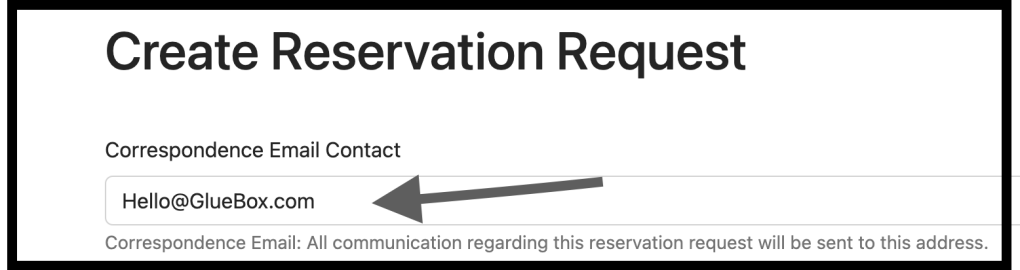
Approach
To locate the field within the Drupal form array, the following steps were undertaken:
Form Inspection: The initial step involved inspecting the HTML of the node/add page to identify the field name and structure. This provided a clue that the field was indeed present on the form and gave us the field's machine name.
Debugging with hook_form_alter: We implemented hook_form_alter in a custom module to inspect the structure of the form array. Initial attempts to print or log the entire form array led to memory exhaustion errors due to the size of the array.
Targeted Logging: Instead of printing the entire array, we resorted to more targeted logging. We looped through the top-level elements of the form array, logging the keys and types of elements to identify potential containers or wrappers for our field.
Recursive Function Implementation: A recursive function was implemented to traverse the form array and search for the field_assigned_user_email field. This was done cautiously to avoid memory limits by checking memory usage before going deeper into the recursion.
Identification of the Container: Through the targeted logging and recursive traversal, we identified that field_assigned_user_email was not directly an input field but was wrapped in a container type with a widget array holding the field.
Correct Array Path: After identifying the widget array, we found that the actual input field was nested within it, and we used the following path to access it:
$form['field_assigned_user_email']['widget'][0]['value']
Setting the Default Value: With the correct path identified, we used the Drupal::token() service to set the default value of the email field to the current user's email address for authenticated users.
Code Implementation
The final implementation in the chas_default_email_value_form_alter function was as follows:
use Drupal\Core\Form\FormStateInterface;
/**
* Implements hook_form_alter() for node_reservation_request_form.
*/
function chas_default_email_value_form_alter(&$form, FormStateInterface $form_state, $form_id) {
if ($form_id == 'node_reservation_request_form' && \Drupal::currentUser()->isAuthenticated()) {
$email_widget = &$form['field_assigned_user_email']['widget'][0];
if (isset($email_widget['value'])) {
$current_user_email = \Drupal::token()->replace('[current-user:mail]', []);
$email_widget['value']['#default_value'] = $current_user_email;
}
}
}
Conclusion
By incrementally debugging the form array and carefully managing resources, we were able to locate the correct array path to set the default value for the field_assigned_user_email. This approach minimized the risk of memory exhaustion and provided a clear path to the solution.